ASP .NET simplified the work of developers with the introduction of a namespace called System.Web.Mail. By using this namespace, developers can easily sending a plain text mail, HTML mail, or sending attachments.
Sending Plain Text Mail
Let’s start with a simple plain text mail first. See the codes below.
using System.Web.Mail;The output of this will look like the screenshot below.
.
.
.
string To = "youremailaddress@yahoo.com";
string From = "webmail@mail.com";
string Subject = "Hi";
string Body = "Hello World!";
SmtpMail.Send(From, To, Subject, Body);
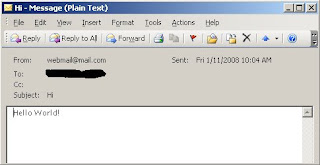
This is very simple since it only uses SmtpMail.Send method in the System.Web.Mail namespace. SmtpMail.Send method contains 4 parameters: From, To, Subject and BodyText.
Sending HTML Mail
In sending a HTML mail, we need to use MailMessage class, which provides a lot of useful properties. Here is a list of the properties:
- Attachments - Used for sending e-mails with attachments
- From - Sender’s email address
- To - Recipient’s email address
- Cc - Recipient’s email address (Carbon Copy)
- Bcc - Recipient’s email address (Blind Carbon Copy)
- Body - Text of the email address
- BodyFormat - Format of an email (Text or HTML)
- Priority - Priority of an email (High, Low, or normal)
- Subject - Title of an email
- Headers - Denotes a collection of acceptable headers (E.g. Reply-To)
- BodyEncoding - Method of encoding an e-mail message (Base64 or UUEncode)
using System.Web.Mail;The output of this will look like the screenshot below.
.
.
.
MailMessage mail = new MailMessage();
mail.To = "youremailaddress@yahoo.com";
mail.Cc = "ccemailaddress@yahoo.com";
mail.From = "webmail@mail.com";
mail.Subject = "Hi, another mail from me";
mail.BodyFormat = MailFormat.Html;
string strBody = "<html><body><b>Hello World</b><br>" +
" <font color=\"red\">A HTML mail</font></body></html>";
mail.Body = strBody;
SmtpMail.Send(mail);
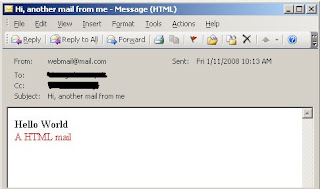
Sending Attachments
As you already seen in the list of MailMessage properties, by sending an attachment, you can use the Attachments property. See the codes below.
using System.Web.Mail;The output of this will look like the screenshot below.
.
.
.
MailMessage mail = new MailMessage();
mail.To = "youremailaddress@yahoo.com";
mail.From = "webmail@mail.com";
mail.Subject = "Attachment from me";
mail.BodyFormat = MailFormat.Text;
mail.Body = "There is an attachment!";
mail.Attachments.Add(new MailAttachment("c:\\Test Message.doc"));
SmtpMail.Send(mail);
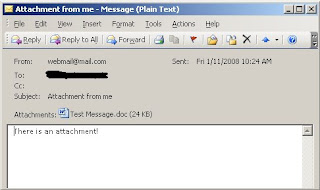
1 comments:
emm.. luv this text )
Post a Comment